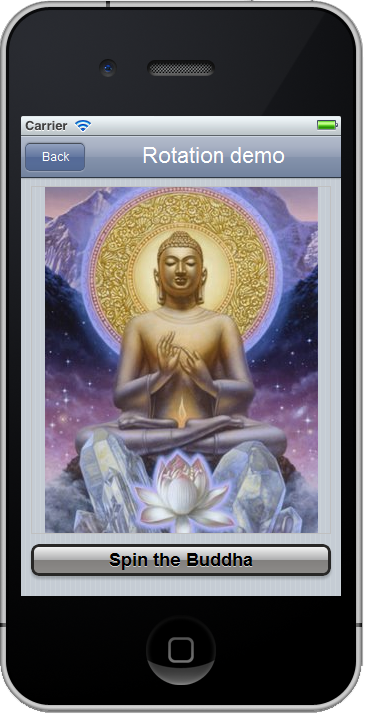
This is not just a picture, but actually a multi layered view. The buddha is a background for an image placeholder. Quite dynamic and neat
Products like Embarcadero Delphi comes with a rich set of controls ready for use in your applications. If we compare the resource demands of these controls today (Delphi XE or XE2) to those that once shipped with Delphi 1 – or Delphi 7 even, the amount of resources a well designed form consumes has grown exponentially over the years.
As the operative system was grown more complex both in terms for functionality, responsiveness, mobility and the developers need for modern features, the amount of methods and auxiliary classes connected to a single control has become very large. As a result, the amount of memory and CPU requirements have grown with it.
The mobile scene
One of my primary concerns when we sat down to talk about OP4JS control library was simply that it would be over-kill to even attempt to emulate the VCL under javascript. In my notes the primary points were:
- The amount of JavaScript generated would exceed the restrictions of these devices
- The HTML5 architecture is incompatible with classical VCL programming
- The controls would be to slow for practical use
Thankfully my worries were unfounded on all accounts, because we have more or less built a VCL clone. It is and has been quite a challenge. As you may know HTML5 introduces a high-level interface onto ordinary html. It is different not so much in terms of syntax, but rather in philosophy, inspired no doubt by the MVC (model view controller) paradigm imposed on the world by Apple computers. In short, the CSS styling represents the “view” part, the actual html tags the “model” and Javascript the controlling factor. Javascript is what provides behavior to otherwise empty, stateless constructs.
Getting these things to even resemble the VCL has been, well, let’s just say I havent played with my kids in a few weeks.
Doing the math
Luckily, the complexity versus efficiency argument is turning in our favour. Delphi developers like myself have a tendency to over-think the actual needs of our customers, and inside our own heads we are always trying to outsmart other programmers that may – or in most cases – may not be going over our code with a fine tooth comb. This can either drive us to excel at what we do or into obsessive nerds that polishes a piece of code so long – that the platform is dead if he ever finishes.
So, once in a while it’s wise to do a sanity check on your code-base (and your own expectations). Fact is that mobile apps are for the most part quite lightweight. If we for sake of argument say that a mobile app has 10 forms (or views if you prefer) and each of these views is populated by 20 controls. Some controls are composite, meaning that they have child controls (like a toolbar which uses a buttons) while other are vanilla, run of the mill stuff like labels and lists. You may also have a database running under the hood as well.
Adaptable and suitable
So an app consisting of 200 javascript objects is really not that huge. It would result in quite a bit of generated code of-course, but the browser chews through this like it was nothing at all. And you wouldn’t even be close to the limitations set down by Apple or Google regarding size. Strange as it may seem – my test app written in Op4JS both loads and executes faster than it’s native counterpart written in C# (which compiles to a single binary on iOS). The only exception being complex graphics (which you would normally use a DIB library for) and heavy-duty number crunching. But those are things you don’t want to have on your phone anyways. It’s possible of-course, but not practical.
To me at least, mobile development is about information and communication and presentation. It’s about getting or storing data and presenting it on a portable device in a practical manner. It’s also about using the format in a way that makes sense to the consumer.
It looks great! Will there be a checkbox or the switch control that we see in many apps such as the Settings? In XE2, there is no switch either and frankly it is too small as is the radio button.
iOS actually has a very cool Checkbox (normal), but people rarely use it. The checkbox you see in my photo above is rendered by Chrome and is not styled yet. As for the switch… yes, i want that to.
But it’s very easy to put it together custom controls yourself. On top of my head, a quick switch could be implemented like this:
[sourcecode language=”delphi”]
Type
//All controls, except canvas based, derive from TW3CustomControl
TSwitchControl = Class(TW3CustomControl)
private
//We want some nice sliding effect of the background
FEffect: TW3HorizontalSlideEffect;
//Just to keep track of the effect
FBusy:Boolean;
//our "checked" value
FValue:Boolean;
//event handler for when the effect is done
Procedure HandleSlideDone(sender:TObject);
Protected
//This is an internal message handler,
// a bit like Delphi’s winapi message handler. It happens before the On[xyz] events..
Procedure CMClick;override;
//We dont override the constructor unless we absolutely have to
procedure InitializeObject;override;
published
// our "checked" value for the world
Property Checked:Boolean read FValue;
End;
//and in the implementation section
procedure TSwitchControl.InitializeObject;
Begin
inherited;
// load an image of the switch, the knob in the center of the image
// It’s blue (checked) on the left side and grey on the right (unchecked)
self.background.Image.fromURL(‘res/switch_mask.png’);
end;
Procedure TSwitchControl.CMClick;
Begin
//If the slider is moving, ignore click
if not FBusy then
Begin
//remember that we are busy
FBusy:=True;
//ok, let’s put webkit to work!
FEffect:=TW3HorizontalSlideEffect.Create;
FEffect.duration:=0.3;
FEffect.fromPos = makePosition(0,0,width,height);
FEffect.toPos = makePosition(-50,0,width,height);
FEffect.onTransitionDone:=HandleSlideDone;
FEffect.execute(self.content);
end;
inherited;
end;
//This method is called when the slide transition is done
Procedure TSwitchControl.HandleSlideDone(Sender:Tobject);
Begin
//Togge the "checked" value
FValue:=w3_ToggleBoolean(FValue);
//release our effect instance
FEffect.free;
//ready for another click!
FBusy:=False;
end;
[/sourcecode]
The above code uses harware accellerated sliding (if possible) through the effect classes. There are more parameters to set naturally (like ease-in/out/linear etc. etc.) but in general terms — it’s very easy to make your own controls. And you are expected to do so in many cases. But if you know how to create a button from code in ordinary Delphi – then you will have no problems what so ever coding new and exciting controls yourself. In fact, we are hoping to get a budding control marked going 🙂