Debugging, exceptions and error handling
Smart has full support for ordinary Object Pascal exceptions, in other words the same system used by both Delphi and free-pascal.
What is an exception?
An exception is simply a signal that something has gone wrong. Under object pascal all exceptions are represented by an exception object, which contains information about the error that has occurred. The most basic exception object simply contains a text string called message, which contains a string representation of the error that occurred. Here is how you raise an exception:
if (something<>whatyouexpect) then raise exception.create('I expected something else');
The exception object is an object like everything else. It is normal to create your own exception objects if your error needs more information than just a string.
Catching exceptions
Catching an exception is done inside a try / except block. If the code inside the block raises an exception then you can safely handle it before either exiting your method or continuing execution:
try DoSomethingThatCanFail; except on e: exception do w3_showmessage('The following error occured:' + e.message); end;
The above code is quite generic. It will catch all errors of type exception (which is all of them). The exception object is made available as the “e” variable. In the above code we display the message (string text) field of the exception.
Being more selective
While catching all types of exception with the same error handler is very effective, there are times when you want to handle specific errors differently. Object Pascal allows you to handle that in the following way:
try DoSomethingThatCanFail; except on e: EW3FirstErrorType do w3_showmessage('A EW3FirstErrorType error occured:' + e.message); on e: EW3SomeOtherErrorType do w3_showmessage('A different error occured:' + e.message); on e: exception do w3_showmessage('All other errors are handled by this one'); end;
Ignoring exceptions
Unless you handle an exception it causes the execution of the current procedure (recursively back to the first caller) to be aborted. In other words, it will stop running your code and propagate the error upwards. So if you don’t handle errors, it will report it to the method that called your code, or exit that and continue until it finds an exception handler. If you simply want to ignore any errors and continue no matter what (not recommended) you can do as such:
try DoSomethingThatCanFail; except on e: exception do; //Ignore any errors and continue end;
Debugging
Since Smart generates javascript designed to run in browsers, the best way to debug is to use a browser. As of writing Smart target’s iOS exclusively (meaning: iPhone and iPad on Safari mobile) and as such the best way to debug is to install and use Safari. Safari has one of the best debuggers built into it.
- Start by downloading and installing Safari on your PC or virtual machine.
- When running Safari for the first time, make sure you set Safari as your main browser
- Go to: Edit -> Options -> Advanced and enable “display developer menu”
To make Smart Mobile Studio use safari rather than it’s built in Chrome instance, do the following:
- In Smart Mobile Studio click on the preferences button, then go to the server tab and disable “use built-in browser to display my apps”. This will force Smart to run your applications in Safari
- When you run your app, go to the safari developer menu and click “show web inspector”
It is also important that you enable debugging info in your project option (Options -> Compiler tab). And of-course it’s equally important that you use try/except sections in your code.
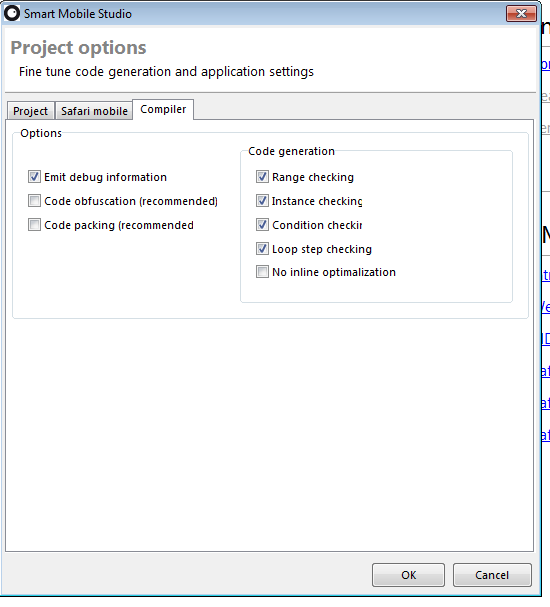
Make sure your project options include debug information, otherwise your exception handling might not make sense